How To Pass Command Line Arguments In Dev C++
Are you ready to be a Master Chef? Unlock new recipes and trophies by passing your cooking courses and exams. Download cooking academy 2 full version gratis. Learn interesting trivia about food while mastering the skills of chopping, kneading, mashing, flipping, frying, and much more. Cooking Academy is the game that places you in the kitchen of a prestigious culinary school - from eggrolls to pancakes, from Ravioli to Creme Brulee, it's up to you to prepare over 50 different recipes. Grab your oven mitts and don your Chef's Hat!
- Command-line arguments in Java are used to pass arguments to the main program. If you look at the Java main method syntax, it accepts String array as an argument. When we pass command-line arguments, they are treated as strings and passed to the main function in the string array argument.
- Apr 22, 2013 Newbie - passing command line arguments in.
- Command-line arguments are given after the name of the program in command-line shell of Operating Systems. To pass command line arguments, we typically define main with two arguments: first argument is the number of command line arguments and second is list of command-line arguments.
- Command Line Arguments In C
- Command Line Arguments Java
- Command Line Commands
- How To Pass Command Line Arguments In Dev C Download
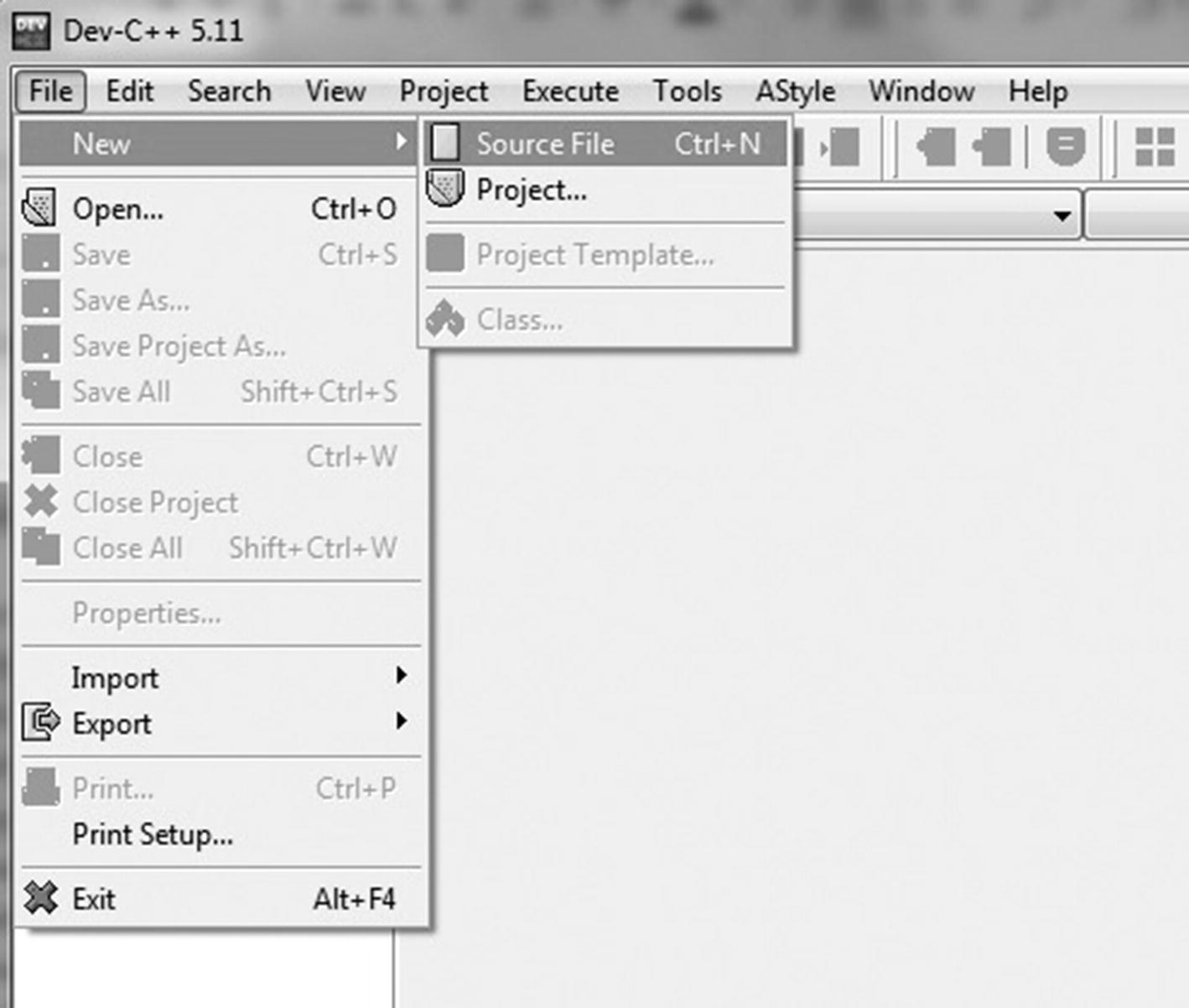
I'm trying to pass arguments to my program so that I can store them into an array. For some reason my IDE/compiler is treating the blank space in between arguments as an argument. I'm using bloodshed, but it doesn't say anything about a seperation character. Can anyone tell me what I'm doing wrong.
-->All C++ programs must have a main
function. If you try to compile a C++ .exe project without a main function, the compiler will raise an error. (Dynamic-link libraries and static libraries don't have a main
function.) The main
function is where your source code begins execution, but before a program enters the main
function, all static class members without explicit initializers are set to zero. In Microsoft C++, global static objects are also initialized before entry to main
. Several restrictions apply to the main
function that do not apply to any other C++ functions. The main
function:
- Cannot be overloaded (see Function Overloading).
- Cannot be declared as inline.
- Cannot be declared as static.
- Cannot have its address taken.
- Cannot be called.
The main function doesn't have a declaration, because it's built into the language. If it did, the declaration syntax for main
would look like this:
Microsoft Specific
If your source files use Unicode wide characters, you can use wmain
, which is the wide-character version of main
. The declaration syntax for wmain
is as follows:
You can also use _tmain
, which is defined in tchar.h. _tmain
resolves to main
unless _UNICODE is defined. In that case, _tmain
resolves to wmain
.
If no return value is specified, the compiler supplies a return value of zero. Alternatively, the main
and wmain
functions can be declared as returning void (no return value). If you declare main
or wmain
as returning void, you cannot return an exit code to the parent process or operating system by using a return statement. To return an exit code when main
or wmain
is declared as void, you must use the exit function.
END Microsoft Specific
Command line arguments
The arguments for main
or wmain
allow convenient command-line parsing of arguments and, optionally, access to environment variables. The types for argc
and argv
are defined by the language. The names argc
, argv
, and envp
are traditional, but you can name them whatever you like.
The argument definitions are as follows:
argc
An integer that contains the count of arguments that follow in argv. The argc parameter is always greater than or equal to 1.
argv
An array of null-terminated strings representing command-line arguments entered by the user of the program. By convention, argv[0]
is the command with which the program is invoked, argv[1]
is the first command-line argument, and so on, until argv[argc]
, which is always NULL. See Customizing Command Line Processing for information on suppressing command-line processing.
The first command-line argument is always argv[1]
and the last one is argv[argc - 1]
.
Note
By convention, argv[0]
is the command with which the program is invoked. However, it is possible to spawn a process using CreateProcess and if you use both the first and second arguments (lpApplicationName and lpCommandLine), argv[0]
may not be the executable name; use GetModuleFileName to retrieve the executable name, and its fully-qualified path.
Microsoft Specific
envp
The envp array, which is a common extension in many UNIX systems, is used in Microsoft C++. It is an array of strings representing the variables set in the user's environment. This array is terminated by a NULL entry. It can be declared as an array of pointers to char (char *envp[]
) or as a pointer to pointers to char (char **envp
). If your program uses wmain
instead of main
, use the wchar_t data type instead of char. The environment block passed to main
and wmain
is a 'frozen' copy of the current environment. If you subsequently change the environment via a call to putenv
or _wputenv
, the current environment (as returned by getenv
or _wgetenv
and the _environ
or _wenviron
variable) will change, but the block pointed to by envp will not change. See Customizing Command Line Processing for information on suppressing environment processing. This argument is ANSI compatible in C, but not in C++.
END Microsoft Specific
Example
The following example shows how to use the argc, argv, and envp arguments to main
:
Parsing C++ command-Line arguments
Command Line Arguments In C
Microsoft Specific
Microsoft C/C++ startup code uses the following rules when interpreting arguments given on the operating system command line:
Arguments are delimited by white space, which is either a space or a tab.
The caret character (^) is not recognized as an escape character or delimiter. The character is handled completely by the command-line parser in the operating system before being passed to the
argv
array in the program.A string surrounded by double quotation marks ('string') is interpreted as a single argument, regardless of white space contained within. A quoted string can be embedded in an argument.
A double quotation mark preceded by a backslash (') is interpreted as a literal double quotation mark character (').
Backslashes are interpreted literally, unless they immediately precede a double quotation mark.
If an even number of backslashes is followed by a double quotation mark, one backslash is placed in the
argv
array for every pair of backslashes, and the double quotation mark is interpreted as a string delimiter.If an odd number of backslashes is followed by a double quotation mark, one backslash is placed in the
argv
array for every pair of backslashes, and the double quotation mark is 'escaped' by the remaining backslash, causing a literal double quotation mark (') to be placed inargv
.
Example
The following program demonstrates how command-line arguments are passed:
The following table shows example input and expected output, demonstrating the rules in the preceding list.
Results of parsing command lines
Command-Line Input | argv[1] | argv[2] | argv[3] |
---|---|---|---|
'abc' d e | abc | d | e |
ab d'e f'g h | ab | de fg | h |
a'b c d | a'b | c | d |
a'b c' d e | ab c | d | e |
END Microsoft Specific
Wildcard expansion
Microsoft Specific
You can use wildcards — the question mark (?) and asterisk (*) — to specify filename and path arguments on the command-line.
Command-line arguments are handled by a routine called _setargv
(or _wsetargv
in the wide-character environment), which by default does not expand wildcards into separate strings in the argv
string array. For more information on enabling wildcard expansion, refer to Expanding Wildcard Arguments.
END Microsoft Specific
Customizing C++ command-line processing
Microsoft Specific
If your program does not take command-line arguments, you can save a small amount of space by suppressing use of the library routine that performs command-line processing. This routine is called _setargv
and is described in Wildcard Expansion. To suppress its use, define a routine that does nothing in the file containing the main
function, and name it _setargv
. The call to _setargv
is then satisfied by your definition of _setargv
, and the library version is not loaded.
Similarly, if you never access the environment table through the envp
argument, you can provide your own empty routine to be used in place of _setenvp
, the environment-processing routine. Just as with the _setargv
function, _setenvp
must be declared as extern 'C'.
Your program might make calls to the spawn
or exec
family of routines in the C run-time library. If it does, you shouldn't suppress the environment-processing routine, since this routine is used to pass an environment from the parent process to the child process.
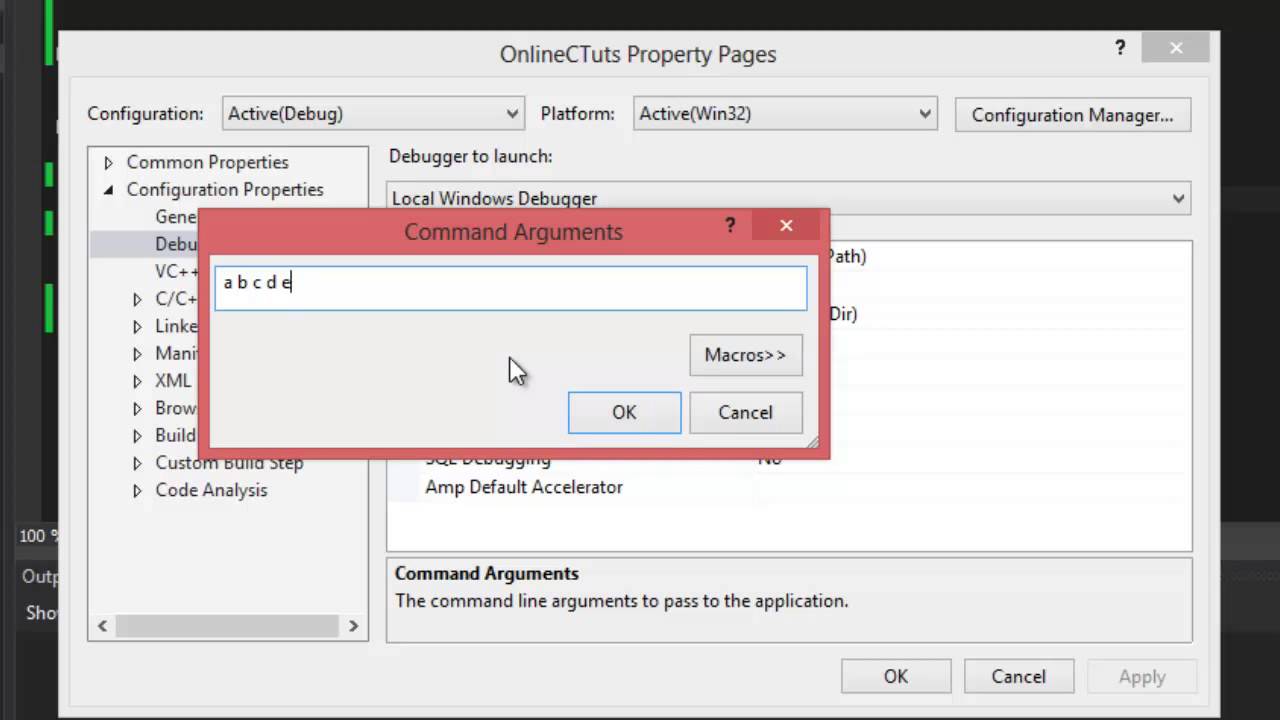
Command Line Arguments Java
END Microsoft Specific
See also
I'm trying to pass arguments to my program so that I can store them into an array. For some reason my IDE/compiler is treating the blank space in between arguments as an argument. I'm using bloodshed, but it doesn't say anything about a seperation character. Can anyone tell me what I'm doing wrong?
I'm doing something like this:
Bob Bill
that should be two parameters, but for some reason it returns three.
- 3 Contributors
- forum 2 Replies
- 897 Views
- 6 Hours Discussion Span
- commentLatest Postby Ancient DragonLatest Post
Dante Shamest
It appears to work for me. The version of my Dev-C++ IDE is 4.9.9.2. One should note that the first parameter is always the name of your program.
This is the program I used for testing.
This picture shows how I passed the parameters.
Command Line Commands
[IMG]http://img438.imageshack.us/img438/724/parameters1fz.th.jpg[/IMG]
How To Pass Command Line Arguments In Dev C Download
And this is my output.